[CS50] Week 1 Review
Week 1
: learning about how to write good code in the programming language C
Lecture Contents
1. Machine Code
-Source Code : human readable
-Machine Code : machine readable, ones and zeros
We should convert source code to machine code using a compiler. A compiler is a very special piece of software.
Source Code -> | Compiler | -> Machine Code |
2. Hello World
*VS Code*
We will be using three commands to write, compile, and run our first program.
code hello.c
make hello
./hello
1) code hello.c : creates a file and allows us to type instructions for this program.
2) make hello : compiles the file from our instructions in C and creates an executable file called hello.
3) ./hello : runs the program called hello.
then, write code as follows :
#include <stdio.h>
int main (void)
{
printf ("hello, world\n");
}
1) printf : a function that can output a line of text.
2) \n : creates a new line after the words hello, world.
3) #include <stdio.h> : tells the compile that you want to use the capabilities of a library called stdio.h, a header file. This allows you, among many other things, to utilize the printf function.
3. Functions
<In Scratch>
: Used say block to display any text on the screen
<In C>
: function printf
printf ("hello, world\n");
4. Variables
<In Scratch>
: we had the ability to ask the user “What’s your name?” and say “hello” with that name appended to it.
<In C>
#include <stdio.h>
int main(void)
{
string answer = get_string("What's your name? ");
printf("hello, %s\n", answer);
}
1) get_string (function) : to get a string from the user
2) %s : a placeholder called a format code that tells the printf function to prepare to receive a string.
3) answer (variable) : is of type string and can hold any string within it.
**There are many data types such as int, bool, char, etc.
-> Notice that numerous errors appear when running make hello because string and get_string are not recognized by the compiler.
#include <cs50.h>
#include <stdio.h>
int main(void)
{
string answer = get_string("What's your name? ");
printf("hello, %s\n", answer);
}
Notice that #include <cs50.h> has been added to the top of your code.
-> Now works!
There are many format codes :
%c
%f
%i
%li
%s
%s is used for string variables. %i is used for int or integer variables.
5. Conditionals
*In C, you can assign a value to an int or integer as follows:
int counter = 0;
*You can add one to counter as follows:
counter = counter + 1;
also as:
counter = counter ++;
Let's use this new knowledge to write some conditionals!
For example, you might want to do one thing if x is greater than y / something else if that condition is not met.
<In Scratch>
: If ~ then, If ~ else ~
<In C>
#include <cs50.h>
#include <stdio.h>
int main(void)
{
int x = get_int("What's x? ");
int y = get_int("What's y? ");
if (x < y)
{
printf("x is less than y\n");
}
}
Flow charts are a way by which you can examine how a computer program functions. Such charts can be used to examine the efficiency of our code :
#include <cs50.h>
#include <stdio.h>
int main(void)
{
int x = get_int("What's x? ");
int y = get_int("What's y? ");
if (x < y)
{
printf("x is less than y\n");
}
else if (x > y)
{
printf("x is greater than y\n");
}
else
{
printf("x is equal to y\n");
}
}
-> You can see the efficiency of our code design decisions.
**string vs char
string : a series of characters
char : a single character
Using char in conditionals :
#include <cs50.h>
#include <stdio.h>
int main(void)
{
// Prompt user to agree
char c = get_char("Do you agree? ");
// Check whether agreed
if (c == 'Y' || c == 'y');
{
printf("Agreed.\n");
}
else if (c == 'N' || c == 'n');
{
printf("Not agreed.\n");
}
}
1) == : something is equal to something else
2) || : or
6. Loops
- How to make computer say 'meow' three times?
1) simply copy + paste
#include <stdio.h>
int main(void)
{
printf("meow\n");
printf("meow\n");
printf("meow\n");
}
=> But we have an opportunity for better design!
2) while loop
#include <stdio.h>
int main(void)
{
int i = 0;
while (i < 3)
{
printf("meow\n");
i++;
}
}
we can even loop forever using the following code:
#include <stdio.h>
#include <cs50.h>
int main(void)
{
while (true)
{
printf("meow\n");
}
}
3) for loop + create my own function
void main(void)
{
printf("meow\n");
}
=> void main (void)
void : the function does not return any values.
(void) : no values are being provided to the function.
#include <stdio.h>
void meow(void);
int main(void)
{
for(int i = 0; i < 3; i++)
{
meow();
}
}
void meow(void)
{
printf("meow\n");
}
=> Notice that meow function is defined at the bottom of the code & the prototype of the function is provided at the top of the code as void meow(void);
#include <stdio.h>
void meow(int n);
int main(void)
{
meow(3);
}
// Meow some number of times
void meow(int n)
{
for (int i = 0, i < n; i++)
{
printf("meow\n");
}
}
=> This is a better design because if you want to change the number of times saying 'meow', you can just change the number of 'n'.
7. Operators and Abstraction
- How to implement a calculator in C.
1) simple way
#include <stdio.h>
#include <cs50.h>
int main(void)
{
// Prompt user for x
int x = get_int("x : ");
// Prompt user for y
int y = get_int("y : ");
// Perform addition
printf("%i\n", x + y);
}
%i : be ready to print the value of x + y, which is int
Mathematical operators included in C :
+ (addition)
- (subtraction)
* (multiplication)
/ (division)
% (for remainder)
2) add function
#include <stdio.h>
#include <cs50.h>
int add(int a, int b);
int main(void)
{
int x = get_int("x: ");
int y = get_int("y: ");
int z = add(x, y);
printf("%i\n", z);
}
int add(int a, int b)
{
int c = a + b;
return c;
}
=> However, do we actually need variable z & c?
It can be further improved as follows:
#include <stdio.h>
#include <cs50.h>
int add(int a, int b);
int main(void)
{
int x = get_int("x: ");
int y = get_int("y: ");
printf("%i\n", add(x,y));
}
int add(int a, int b)
{
return a + b;
}
=> We can put add function in printf function.
3) truncation : truncates a double value after the decimal point and gives the integer part as the result.
#include <stdio.h>
#include <cs50.h>
int main(void)
{
long x = get_long("x: ");
long y = get_long("y: ");
printf("%li\n", x + y);
}
8. Linux and the Command Line
- Linux : an operating system that is accesible via the command line in the terminal window in VS Code.
- cd : for changing our current directory
- cp : for copying files and directories
- ls : for listing files in a directory
- mkdir : for making a directory
- mv : for moving (renaming) files and directories
- rm : for removing (deleting) files
- rmdir : for removing (deleting) directories
9. Mario
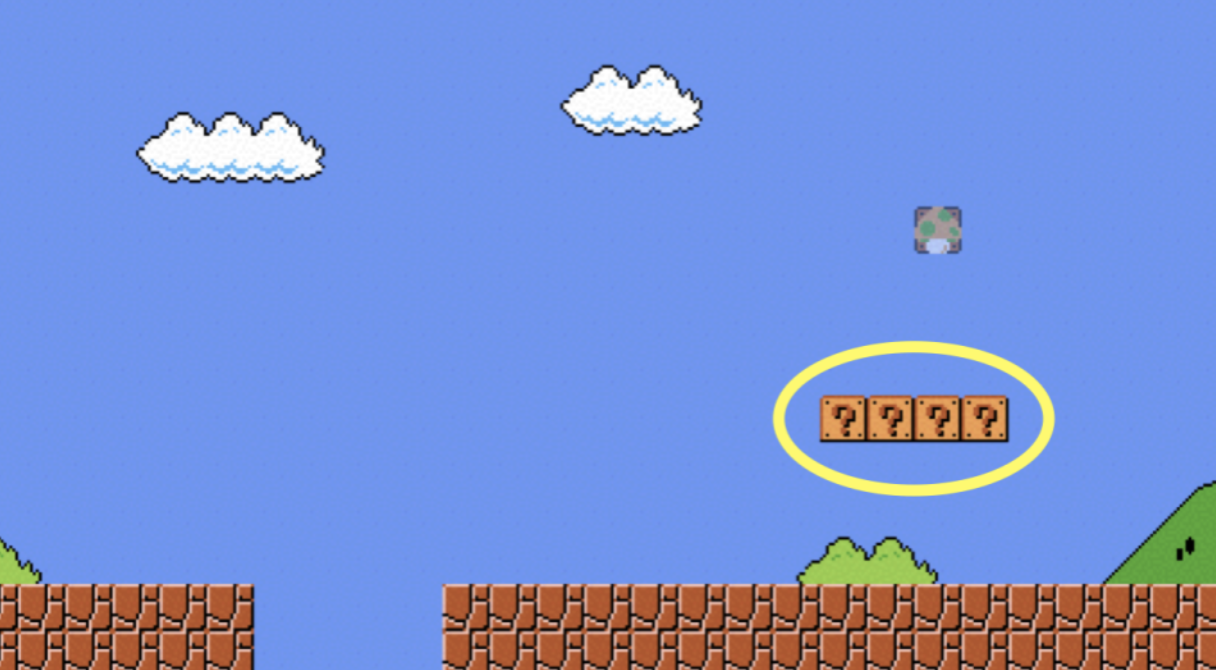
1) How to represent these four horizontal blocks?
#include <stdio.h>
int main(void)
{
for (int i = 0; i < 4; i++)
{
printf("?");
}
printf("\n");
}
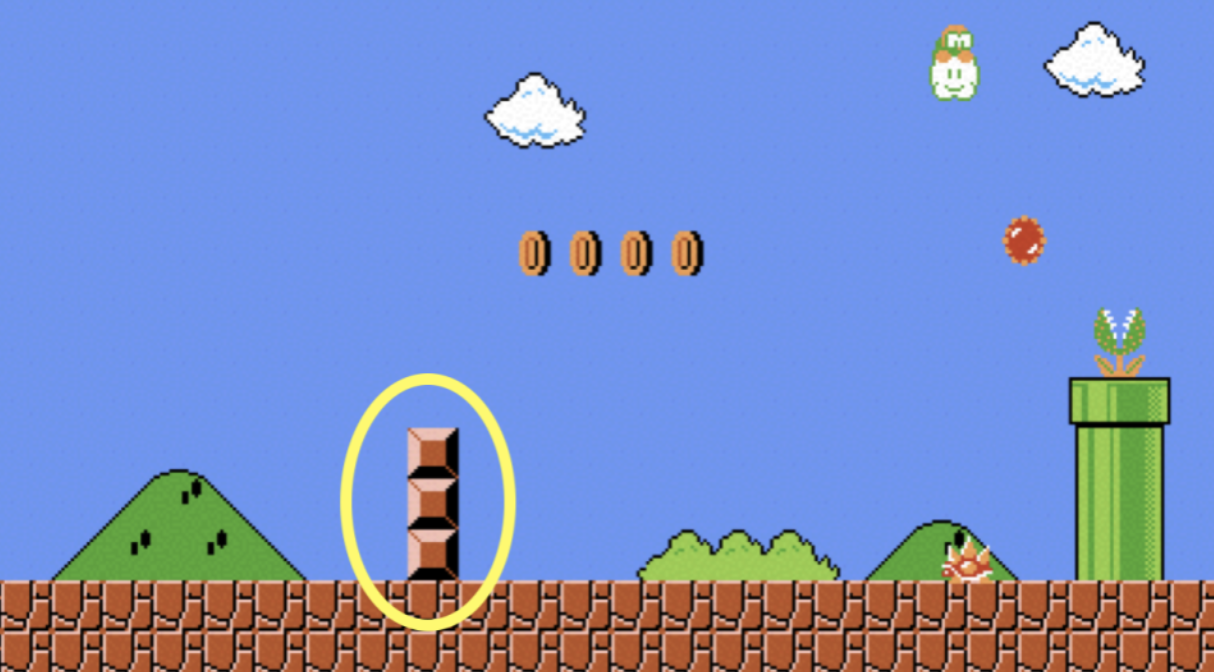
2) How to represent these three vertical blocks?
#include <stdio.h>
int main(void)
{
for (int i = 0, i < 3; i++)
{
printf("#\n");
}
}
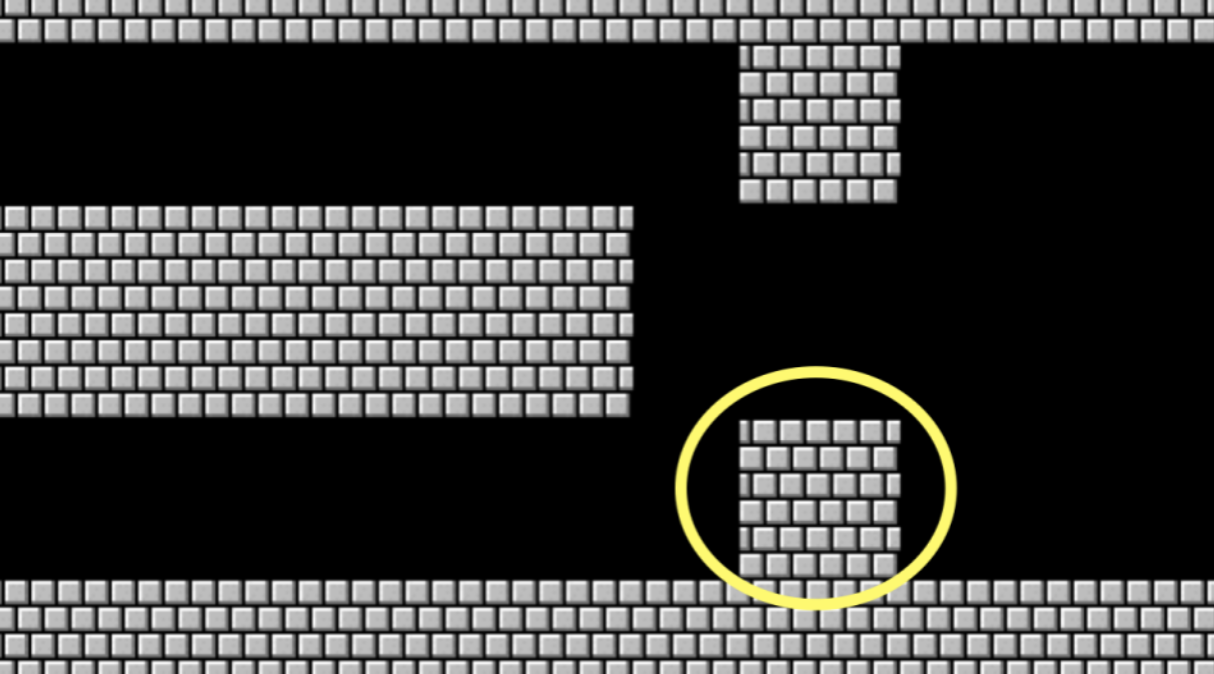
3) How to make a three-by-three group of blocks?
#include <stdio.h>
int main(void)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
printf("#");
}
printf("\n");
}
}
=> ###
###
###
Notice that one loop is inside another.
4) What if we want to ensure the number of bloks to be constant = unchangeable?
#include <stdio.h>
int main(void)
{
const int n = 3;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
printf("#");
}
printf("\n");
}
}
=> now n is a constant, which means it can never be changed.
5) How to prompt the user for the size of the grid?
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int n = get_int("Size: ");
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
printf("#");
}
printf("\n");
}
}
=> get_int
★ We can protect our program form bad behavior by checking to make sure the user's input satisfies our needs.
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int n;
do
{
n = get_int("Size: ");
}
while (n < 1);
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
printf("#");
}
printf("\n");
}
}
=> Now the user will be continuously prompted for the size until the user's input is 1 or greater.
10. Comments
-where you leave explanatory remarks to yourself and others.
-each comment is a few words or more, providing the reader an opportunity to understand what is happening in a specific block of code.
-form : // comment
#include <cs50.h>
#include <stdio.h>
int main(void)
{
//Prompt user for positive integer
int n;
do
{
n = get_int("Size: ");
}
while (n < 1);
// Print a n-by-n grid of bricks
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
printf("#");
}
printf("\n");
}
}
11. Types
-Types refer to the possible data that can be stored within a variable.
Ex) char : a single character like 'a' or '2'.
-Types are very important because each type has specific limits.
Ex) the highest value of int : 4294967295
- bool : a Boolean expression of either true or false
- char : a single character like 'a' or '2'
- double : a floating-point value with more digits than a float
- float : a floating-point value, or real number with a decimal value
- int : integers up to a certain size, or number of bits
- long : integers with more vits, so they can count higher than an int
- string : a string of characters