Section은 Lecture과 Pset 사이의 간극을 줄여주는 추가 강의 세션입니당
Pset 풀기 전에 이걸 듣고 했다면 더 쉬웠을까요..
아무튼 중요하다고 생각되는 부분들만 메모해놓겠음!!
1. Variable : name for some value that can change
int calls = 3;
int : (data) type / calls : name / 3 : value / = : assignment operator
2. Data Types
-Why do we need to decide which data types to use?
: It would determine what we can make the variable do!
<Truncation>
int calls = 4;
calls += 1;
calls -= 2;
calls *= 3;
calls /= 2;
-In this case, int calls = 4;
because we're storing it in integer!
(float calls = 4.5;)
3. Placeholder
int main(void)
{
string first_name = get_string("What is your first name?");
string last_name = get_string("What is your last name?");
printf("Hello, %s %s\n", first_name, last_name);
}
=> There can be multiple placeholders if you need!
Ex) 5th line : %s %s
4. While Loops
int j = 0;
while (j < 4)
{
printf("#");
j++;
}
printf("\n");
=> ####\n
5. For Loops
for (int j = 0; j < 4; j++);
{
printf("#");
}
printf("\n");
=> ####\n
6. Loop in Loop (Don't be afraid to put loop in loop!)
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
printf("#");
}
printf("\n");
}
=> ####\n
####\n
####\n
####\n
7. Mario (Pset 1)
#include <stdio.h>
#include <cs50.h>
//List Function Prototype at the top (because C reads from top to bottom)
void print_row(int length);
int main(void)
{
int height = get_int ("Height: ");
for (int i =0; i < height; i++)
{
print_row(i + 1); //get the input of height from the user and use my own function
}
//Creating my own function called print_row
void print_row(int length)
{
for (int i = 0; i < length; i++)
{
printf("#");
}
printf("\n");
}
★ You can create your own function (Here, for example, print_row())
-> But remember to list that function prototype at the top so that C can read it from top to bottom
★ print_row (i + 1) : enables you to print something like this:
#
##
###
...
★ How do we change it from left-lined to right-lined? Like this:
# #
## -> ##
### ###
=> Let's look at the relationship between 4 variables (height, row, column, and space)
height | row | space | column |
Ex) 4 | 0 | 3 | 1 |
1 | 2 | 2 | |
2 | 1 | 3 | |
3 | 0 | 4 |
★ Space = height - row - 1
The result being like:
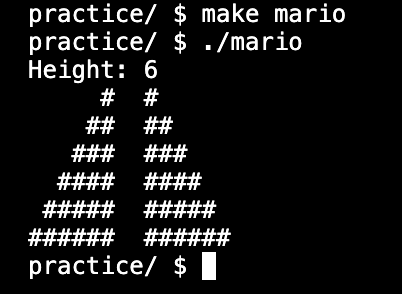
'CS50' 카테고리의 다른 글
[CS50] Week 2 Section (0) | 2024.09.24 |
---|---|
[CS50] Week 2 Review (2) | 2024.09.23 |
[CS50] Pset 1 : Credit (1) | 2024.09.13 |
[CS50] Week 1 Review (1) | 2024.09.10 |
[CS50] Week 0 Review (2) | 2024.08.28 |